1st Step: Create a Navbar Routes Component
“use client”
import React from ‘react’
const guestRoutes = [
{
icon: MdDashboard,
label: “home”,
href: “/doctordashboard”
},
{
icon: FaCartShopping,
label: ” setavailability “,
href: “/ setavailability “
},
]
const NavbarRouts = () => {
const routes = guestRoutes;
return (
<div className=’flex text-gray-600 flex-col w-full’>
{
routes.map((route) => (
<NavbarItem
key={route.href}
icon={route.icon}
label={route.label}
href ={route.href}
/>
))
}
</div>
)
}
export default NavbarRoutes;
2nd Step: Creates a Navbar Item Component
“use client”
import React from ‘react’;
import { IconType } from ‘react-icons’;
import { usePathname, useRouter } from ‘next/navigation’;
interface NavbarItemProps {
icon: IconType;
label: string;
href: string;
}
const NavbarItem = ({ icon: Icon, label, href }: NavbarItemProps) => {
const pathname = usePathname();
const router = useRouter();
console.log(pathname, “docotor”)
const isActive =
(pathname === ‘/’ && href === ‘/’) ||
pathname === href ||
pathname?.startsWith(`/${href}`);
const onClick = () => {
router.push(href);
// router.push(`doctor/${href}`);
};
return (
<div>
<button
onClick={onClick}
type=”button”
className={`flex items-center text-gray-400 text-sm py-4 p-5 space-x-2 ${
isActive
? ‘ w-full cursor-pointer border-r-2 border-sky-400 bg-sky-50/90 font-semibold font-sans transition duration-300 text-sky-500 text-sm’
: ”
}`}
>
<div className={`text-gray-400 ${isActive ? “text-sky-500″ :””}`}>
<Icon size={20} /> {/* Adjust the size as needed */}
</div>
<div >
{label}
</div>
</button>
</div>
);
};
export default Navbar Item;
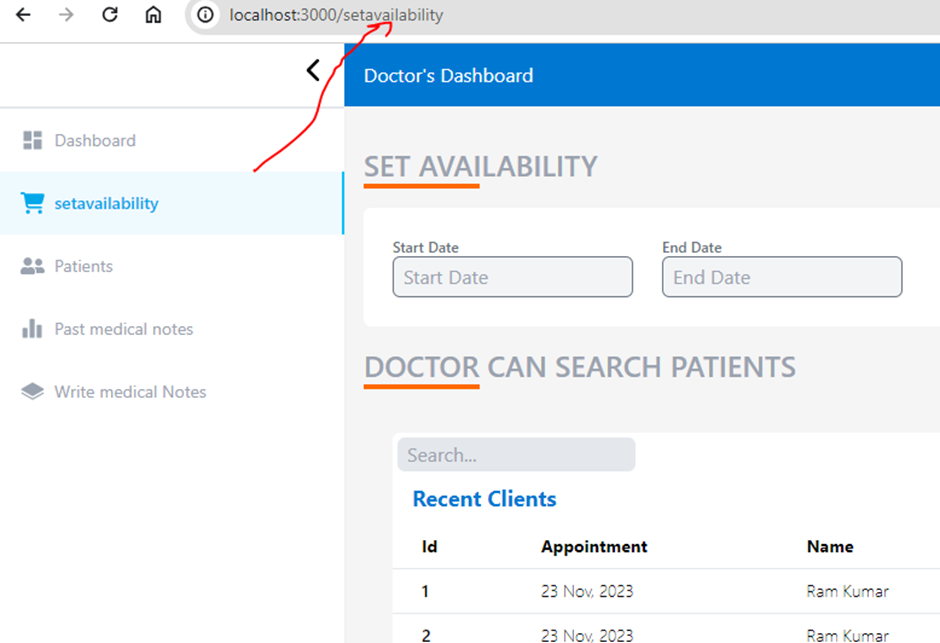